My third REST API
Idea
So that know how to get data from the domino directory, how about if we can change some field value in the domino directory? Let’s change the email property of a certain user !
Configuration
Since we will protect the execution of the route with an api key, we can adjust the ACL (Access Control List) of the myfirst.nsf and add Anonymous as reader.
Reopen XRest API Routes in the notes designer
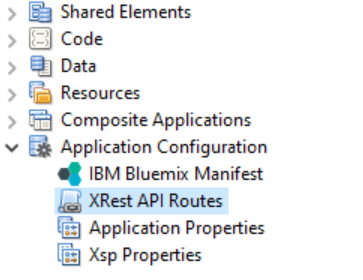
Copy our third route and paste it into the groovy configuration document at the end.
def wgc_api_key = '111-222-333-444-555';
router.POST('updateuser/{id}') {
strategy(DOCUMENT_BY_UNID) {
keyVariableName('id')
databaseName('names.nsf')
runAsSigner(true)
form('Person')
}
allowedAccess { context->
def key = context.getRequest().getHeader('wgc-api-key');
return key == wgc_api_key;
}
events PRE_SAVE_DOCUMENT: {
context, document ->
nsfHelp = context.getNSFHelper()
nsfHelp.computeWithForm(document)
}
description '<b>Domino Directory -> update person record</b>'
mapJson 'UNID', json:'unid', type:'STRING', isformula:true, formula:'@Text(@DocumentUniqueID)', readonly:true
mapJson 'FirstName', json:'firstname', type:'STRING'
mapJson 'LastName', json:'lastname', type:'STRING'
mapJson 'InternetAddress', json:'email', type:'STRING'
}
The groovy configuration looks similar to this. Save, close and build.
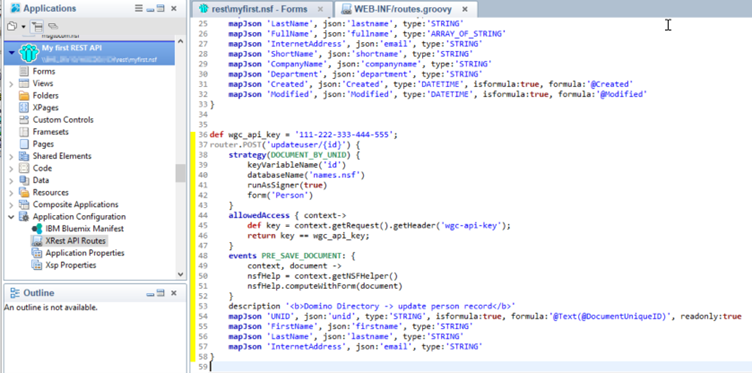
Further explanations
router.POST
form('Person')
runAsSigner(true)
wgc-api-key
We would like to submit data
Enhance the strategy with the form property
Execution occurs in the context of the groovy doc signer
Execution of the route is protected by using a key
The configuration form('Person') is taken into consideration when creating a new document
The execution of the route is protected using a key. In Line 36, we define the key. Attention, the variable contains underscores.
Via postman / header, you define the key: wgc-api-key (Attention: dashes, no underscores !!!)
In the lines 44 - 47 we check if the keys match and if the do, access is allowed.
Testing
The URL to test the third route is:
http://localhost/rest/myfirst.nsf/xsp/.xrest/updateuser/545C406233196751C1258954003E93D8
The unid of the document we want to change is contained in the url.
And the name and value of the property we want to change, we have to submit in the body as a JSON payload.
{
"email": "smartNSF@webgate.biz"
}
Don't forget to switch the postman configuration to POST as we want to submit a new value for the email address.
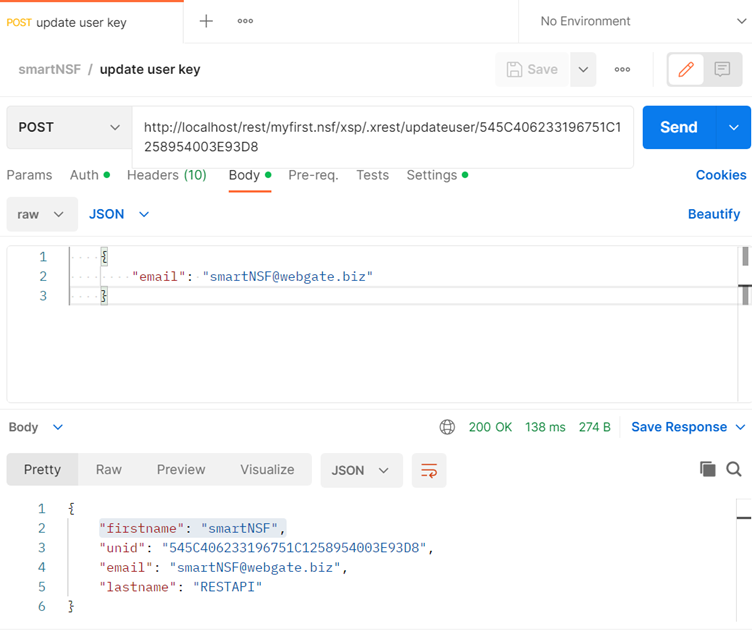
The Body displays already the answer we receive from the REST API call. Notice that the email has been changed already.
What have we learned?
- We can modify records in the notes application.
- We protect the execution using an API key.
- We can define properties as readonly, which should not be changed:
mapJson "UNID", json:'unid', type:'STRING', isformula:true, formula:'@Text(@DocumentUniqueID)', readonly:true - By the way, with the same route we can also create new documents. Just replace the unid with @new
http://localhost/rest/myfirst.nsf/xsp/.xrest/updateuser/@new - And have you noticed that we executed a compute with form before saving the record?
events PRE_SAVE_DOCUMENT: {
context, document ->
nsfHelp = context.getNSFHelper()
nsfHelp.computeWithForm(document)
}
Conclusion
As we have demonstrated, turning any notes application into a REST Service is pretty easy. You have the possibilities to read data as well as to create / update data. You are even capable of CRUD operations (Create, Read, Update, Delete) against an attachment. And don’t forget, we are still in a notes application, means you can enhance your solution with a logging feature for example.
That opens up quite a lot of possibilities. Modernizing your applications can be done in two steps to reduce the risk or just split the project into smaller junks: First the front end and in a second step your back end. Migrations might get easier because you can provide your data in a standardized way.
Where to go from now? If you need more examples, then have a look in the sources in the demo folder. There is an on disk project from where you can build a discussion.nsf as a sample application.
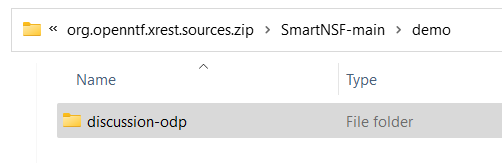
We wish you success in building your REST API's.