Authorization
We have several possibilities to protect our routes from unauthorized execution.
API Key
Protect the execution of the routes using an API key.
Define Anonymous as Reader in the ACL.
Route definition code for API Key
def wgc_api_key = '111-222-333-444-555';
router.GET('user/{id}') {
strategy(DOCUMENT_BY_UNID) {
keyVariableName('id')
databaseName('names.nsf')
runAsSigner(true)
}
allowedAccess { context->
def key = context.getRequest().getHeader('wgc-api-key');
return key == wgc_api_key;
}
description '<b>Domino Directory -> get one user by name</b>'
mapJson 'UNID', json:'unid', type:'STRING', isformula:true, formula:'@Text(@DocumentUniqueID)'
mapJson 'FirstName', json:'firstname', type:'STRING'
mapJson 'LastName', json:'lastname', type:'STRING'
mapJson 'FullName', json:'fullname', type:'ARRAY_OF_STRING'
mapJson 'InternetAddress', json:'email', type:'STRING'
mapJson 'ShortName', json:'shortname', type:'STRING'
mapJson 'CompanyName', json:'companyname', type:'STRING'
mapJson 'Department', json:'department', type:'STRING'
mapJson 'Created', json:'Created', type:'DATETIME', isformula:true, formula:'@Created'
mapJson 'Modified', json:'Modified', type:'DATETIME', isformula:true, formula:'@Modified'
}
Testing using postman
Add the Authentication Type API Key to the header and set the name of the key and its value.
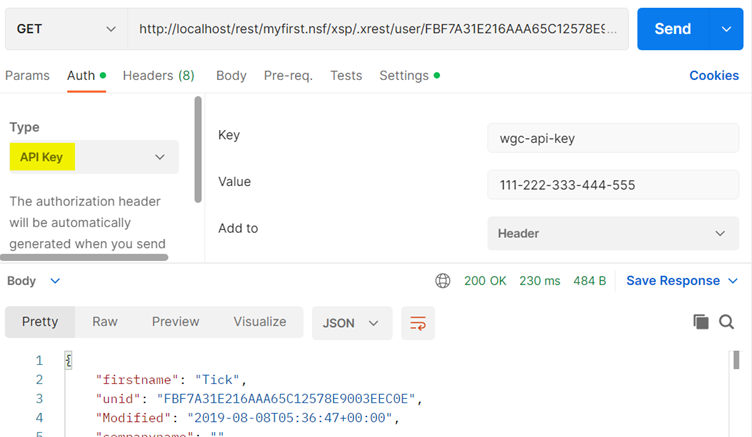
Authorization Endpoint
If you are doing the authorization using an LTPA token, you need to remove the runAsSigner(true) option.
Route definition code for Authorization Endpoint
router.authorizationEndpoint {
additionalDirectories 'folder/seconddirectory.nsf'
publicKey "-----BEGIN PUBLIC KEY-----"+
"111"+
"222"+
"333"+
"444"+
"555"+
"-----END PUBLIC KEY-----"
onUserNotFound {
context,userObject->
println('User not found')
}
Header 'smartnsf-auth'
ssoDomain 'hostname.domain.ch'
}
RunAsSigner
Execution runs with the name of the user which signed the groovy document.
AccessPermission '[Role]'
Protect the execution of the routes using an ACL role.
Define the role “ExecRoutes” and associate the required user to the just created role.
Route to protect the execution using an ACL role.
router.GET('users') {
strategy (DOCUMENTS_BY_VIEW) {
databaseName('names.nsf')
viewName('People')
}
accessPermission '[ExecRoutes]'
description '<b>Domino Directory -> get all users</b>'
mapJson 'UNID', json:'unid', type:'STRING', isformula:true, formula:'@Text(@DocumentUniqueID)'
mapJson 'FirstName', json:'firstname', type:'STRING'
mapJson 'LastName', json:'lastname', type:'STRING'
mapJson 'FullName', json:'fullname', type:'ARRAY_OF_STRING'
mapJson 'InternetAddress', json:'email', type:'STRING'
}